How to Change the Speed of a Servo Motor
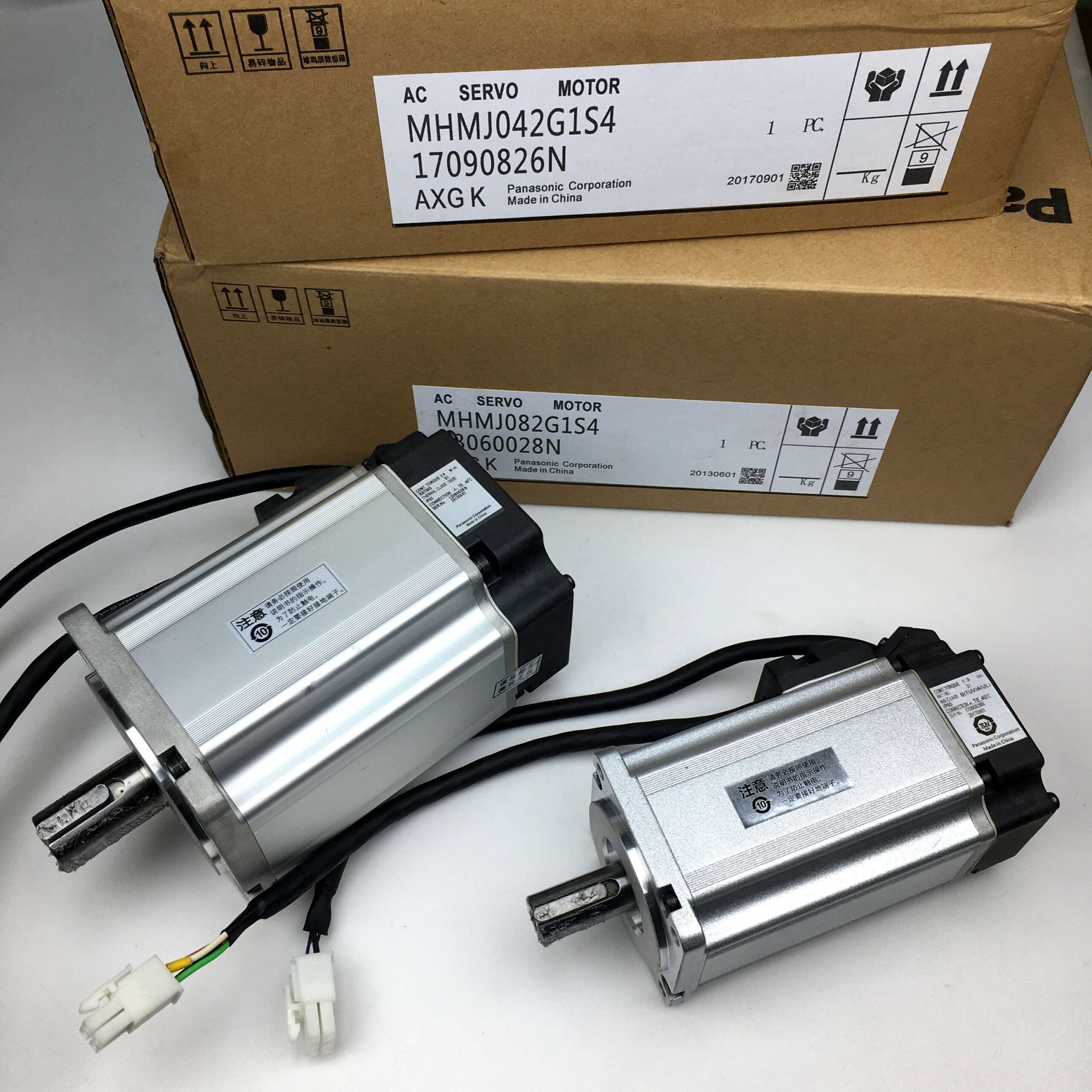
Changing the speed of a servo motor is a fundamental skill for anyone involved in robotics, automation, or electronics projects. Whether you’re working with an Arduino board or a more complex servo drive system, understanding how to adjust your servo motor’s speed can enhance the performance and precision of your applications. This guide provides a comprehensive overview of the methods and tools needed to effectively control and modify servo motor speed, ensuring your projects run smoothly and efficiently.
Methods to Change Servo Speed
There are multiple methods to adjust the speed of a servo motor, each suited to different applications and levels of complexity. Below are some of the most common techniques:
1. Adjusting Pulse Width
Servo motors are typically controlled using Pulse Width Modulation (PWM) signals. By varying the width of these pulses, you can control the speed and position of the servo.
2. Using a Servo Controller
Dedicated servo controllers provide more advanced control over servo motors, allowing for precise speed adjustments and feedback integration.
3. Implementing PID Control
Proportional-Integral-Derivative (PID) controllers can be used to dynamically adjust the speed of servo motors based on real-time feedback, ensuring accurate and stable performance.
4. Software-Based Control with Microcontrollers
Microcontrollers like Arduino offer flexible programming options to control servo speed through software, enabling custom speed profiles and dynamic adjustments.
Each of these methods offers different levels of control and complexity, allowing you to choose the best approach for your specific needs.
Using Pulse Width Modulation (PWM)
Pulse Width Modulation is the most common method for controlling servo motors. PWM involves sending a series of electrical pulses to the servo, with the width of each pulse determining the motor’s position and speed.
How PWM Controls Speed
- Pulse Width Variation: By increasing or decreasing the pulse width, you can make the servo motor move faster or slower.
- Frequency Adjustment: Changing the frequency of the PWM signal can also affect the speed and responsiveness of the servo.
Example:
A standard servo motor expects a PWM signal with a period of 20 milliseconds. A pulse width of 1.5 milliseconds typically sets the servo to its neutral position. Increasing the pulse width moves the servo in one direction, while decreasing it moves the servo in the opposite direction.
Implementing PWM with Arduino
Using an Arduino board, you can easily generate PWM signals to control your servo motor’s speed. Libraries like Servo.h
simplify this process, allowing you to write code that adjusts the pulse width based on your requirements.
#include <Servo.h>
Servo myServo;
int pos = 0;
void setup() {
myServo.attach(9); // Attach servo to pin 9
}
void loop() {
for (pos = 0; pos <= 180; pos += 1) { // Sweep from 0 to 180 degrees
myServo.write(pos);
delay(15); // Adjust delay to change speed
}
for (pos = 180; pos >= 0; pos -= 1) { // Sweep back
myServo.write(pos);
delay(15); // Adjust delay to change speed
}
}
In this example, adjusting the delay
value changes the speed at which the servo moves.
Controlling Speed with Arduino
Arduino boards offer a versatile platform for controlling servo motor speed. By leveraging the Servo.h
library and PWM signals, you can create custom speed profiles and respond to real-time feedback.
Setting Up Your Arduino
- Connect the Servo: Attach the servo motor’s control wire to a PWM-capable pin on the Arduino (e.g., pin 9).
- Install the Servo Library: Ensure the
Servo.h
library is included in your Arduino IDE. - Write the Control Code: Use the library functions to set the servo’s position and adjust the speed.
Example: Dynamic Speed Control
#include <Servo.h>
Servo myServo;
int pos = 0;
int speed = 10; // Adjust this value to change speed
void setup() {
myServo.attach(9);
}
void loop() {
for (pos = 0; pos <= 180; pos += 1) {
myServo.write(pos);
delay(speed); // Lower value = faster movement
}
for (pos = 180; pos >= 0; pos -= 1) {
myServo.write(pos);
delay(speed);
}
}
By modifying the speed
variable, you can control how quickly the servo moves between positions.
Adjusting Servo Parameters
Fine-tuning servo parameters can significantly impact the motor’s speed and performance. Key parameters include:
1. Pulse Width Range
The range of pulse widths (typically 1ms to 2ms) determines the servo’s movement range. Adjusting this range can influence the speed and precision of the motor.
2. Servo Gain
Servo gain settings in the controller can affect how aggressively the motor responds to position changes, impacting speed and stability.
3. Gear Ratio
The gear ratio in a servo motor’s gearbox affects torque and speed. A higher gear ratio increases torque but reduces speed, while a lower ratio does the opposite.
4. Voltage Supply
Providing a higher voltage can increase the servo’s speed and torque, but it’s essential to stay within the manufacturer’s recommended specifications to avoid damage.
Table: Common Servo Parameters and Their Effects
Parameter | Effect on Servo Motor Speed |
---|---|
Pulse Width Range | Adjusts movement range and speed |
Servo Gain | Controls responsiveness and speed |
Gear Ratio | Balances torque and speed |
Voltage Supply | Increases or decreases speed |
Understanding and adjusting these parameters allows for precise control over your servo motor’s performance.
Common Problems and Solutions
Controlling the speed of a servo motor can present several challenges. Here are some common issues and their solutions:
1. Overheating
Issue: Prolonged high-speed operation can cause the servo motor to overheat.
Solution: Implement cooling solutions such as heat sinks or fans. Also, ensure that the servo is not being overdriven beyond its specified limits.
2. Inconsistent Movement
Issue: Servo motors may exhibit jerky or inconsistent movement due to signal noise or inadequate power supply.
Solution: Use shielded cables to reduce noise and ensure a stable power supply. Incorporating capacitors can also help smooth out power fluctuations.
3. Limited Speed Range
Issue: Some servo motors have a limited speed range, making it difficult to achieve desired speeds.
Solution: Choose a servo motor with a suitable gear ratio and voltage rating for your application. Alternatively, use a servo controller that allows for more precise speed adjustments.
4. Latency in Control
Issue: Delays in processing PWM signals can result in sluggish or unresponsive servo movements.
Solution: Optimize your control code for efficiency and consider using a faster microcontroller if necessary. Reducing the complexity of the control loop can also help.
By addressing these challenges, you can ensure smooth and reliable speed control of your servo motors.
Choosing the Right Servo Motor
Selecting the appropriate servo motor is crucial for achieving optimal speed control and performance. Consider the following factors when choosing a servo motor:
1. Torque Requirements
Ensure the servo motor can provide sufficient torque for your application. High-torque applications may require servo motors with larger gear ratios.
2. Speed Specifications
Different servo motors offer varying speed ranges. Choose a motor that meets the speed requirements of your project, balancing it with torque needs.
3. Control Interface
Consider the type of control interface you need, such as PWM, analog, or digital control. Ensure compatibility with your existing systems, like Arduino boards.
4. Size and Weight
The physical size and weight of the servo motor should be compatible with your project’s design constraints.
5. Voltage and Power Supply
Verify that the servo motor operates within the voltage range of your power supply. Providing adequate power is essential for maintaining consistent speed and torque.
Example: Choosing Between Yaskawa and Panasonic Servo Motors
Yaskawa servo motors, such as the SGD7S-3R8A00A, are known for their high precision and durability, making them suitable for industrial applications. Panasonic servo motors, on the other hand, might offer different features or price points that better fit specific project needs.
FAQs
1. What is the difference between a servo motor and a regular DC motor?
A servo motor includes a feedback system, such as an encoder or potentiometer, that allows for precise control of position and speed. In contrast, a regular DC motor lacks this feedback, making it less accurate for applications requiring precise movements.
2. Can I control the speed of any servo motor?
While most servo motors allow for speed control via PWM signals, the range and precision of speed adjustments depend on the specific motor’s design and controller. Always refer to the manufacturer’s specifications for optimal results.
3. How does gear ratio affect servo motor speed?
A higher gear ratio increases the torque but reduces the speed of the servo motor, while a lower gear ratio decreases the torque and increases the speed. Selecting the appropriate gear ratio is essential for balancing speed and torque based on your application’s needs.
4. What tools do I need to change the speed of a servo motor?
To change the speed of a servo motor, you typically need a microcontroller (like an Arduino board), a servo motor controller, appropriate wiring, and possibly additional components like resistors or capacitors to stabilize the signal.
5. Is it possible to reverse the direction of a servo motor?
Yes, by adjusting the PWM signal’s pulse width, you can control the servo motor’s direction of movement. This allows for bidirectional control based on your project’s requirements.
Conclusion
Changing the speed of a servo motor is a vital aspect of many technical projects, offering enhanced control and precision. By understanding the fundamentals of servo motors, implementing effective control methods like PWM and Arduino-based systems, and addressing common challenges, you can achieve optimal performance in your applications. Selecting the right servo motor and fine-tuning its parameters ensures that your projects run smoothly and efficiently.
Key Takeaways:
- Servo Motors: Essential for precise control in various applications.
- Speed Control Methods: PWM, servo controllers, PID control, and microcontroller-based solutions.
- Parameter Adjustment: Pulse width, servo gain, gear ratio, and voltage are crucial for speed management.
- Challenges: Overheating, inconsistent movement, limited speed range, and control latency can be mitigated with proper strategies.
For more information on high-quality servo motors and controllers, visit our Servo Motor Store and explore our range of Yaskawa Servo Motors.
Yaskawa Servo Motor
Panasonic Servo Motor