How to Control a Servo Motor Using a Microcontroller
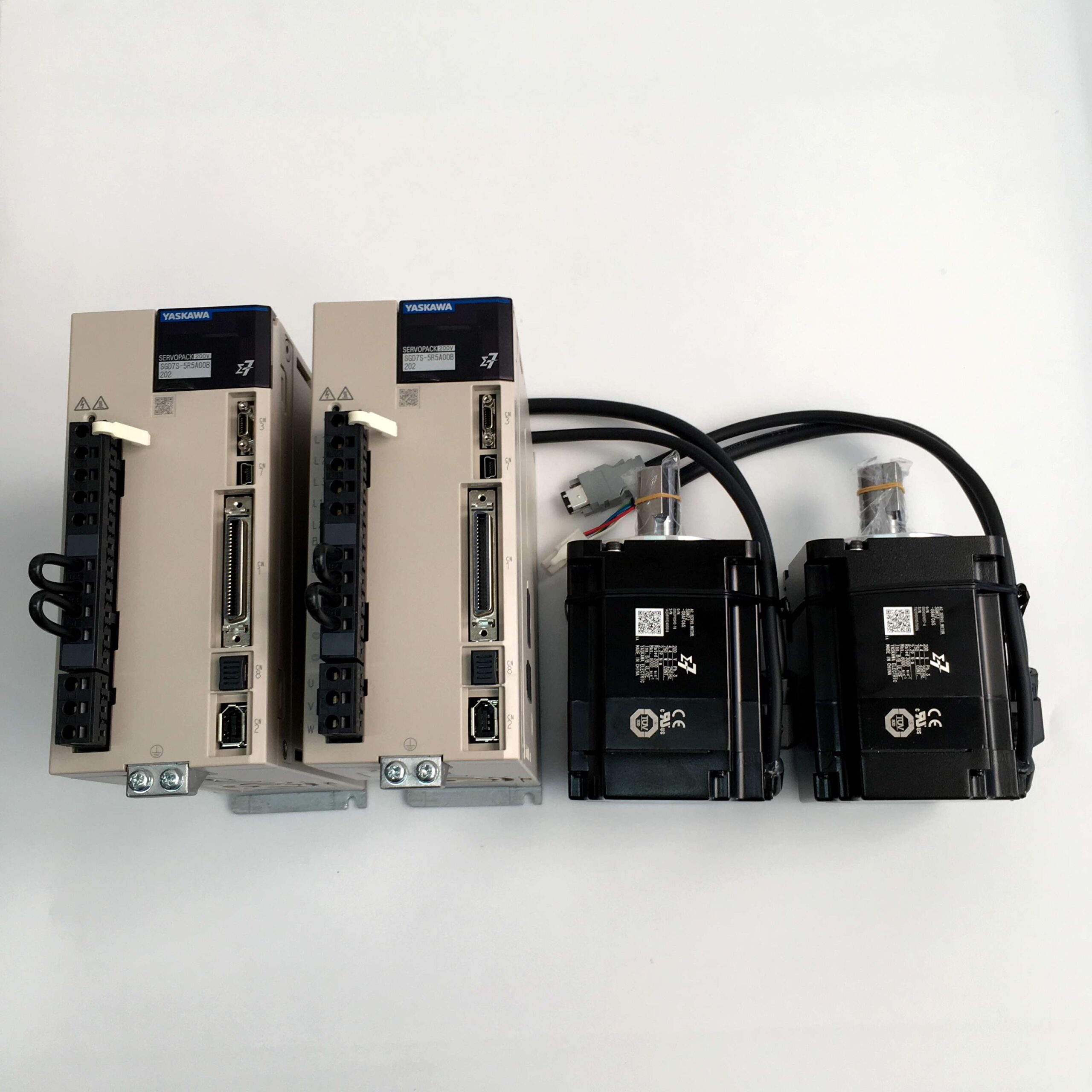
Servo motors are a critical component in robotics and automation systems, allowing precise movement control over position, rotation, and speed. Microcontrollers, on the other hand, provide the intelligence to control such precise movements. This article will provide a detailed guide on how to control a servo motor using a microcontroller, offering a clear, step-by-step approach suitable for beginners and professionals alike.
Servo motors are controlled by sending a Pulse Width Modulation (PWM) signal from a microcontroller, such as Arduino or PIC, to achieve desired rotation angles. Let’s dive into how this all works, the necessary components, and steps to implement such a system.
How PWM Controls a Servo Motor
PWM (Pulse Width Modulation) is a crucial part of servo motor control. The microcontroller generates PWM pulses to determine the angular position of the servo. The pulse width varies between 1ms to 2ms, where:
- A 1ms pulse represents 0 degrees.
- A 1.5ms pulse represents 90 degrees.
- A 2ms pulse represents 180 degrees.
By varying the pulse width, you can achieve precise positioning. The microcontroller reads the input commands, processes them, and sends the appropriate PWM signals to control the motor’s position.
Components Needed for Controlling a Servo Motor
To control a servo motor using a microcontroller, you will need the following components:
- Microcontroller (Arduino, PIC, or ESP32)
- Servo Motor (Standard RC servo motor)
- Power Supply
- Breadboard and Jumper Wires
- PWM Signal Generator (In-built in microcontroller)
Component | Description |
---|---|
Microcontroller | Generates control signals |
Servo Motor | Converts control signals to motion |
Power Supply | Provides electrical power |
Breadboard | For easy circuit connections |
Jumper Wires | Connect microcontroller to servo motor |
Tip: Make sure your power supply voltage matches the requirements of both the microcontroller and servo motor to avoid damaging components.
Step-by-Step Guide to Control a Servo Motor Using Microcontroller
Step 1: Setting Up the Hardware
Connect the Servo Motor to the Microcontroller
- Power Connection: Connect the VCC pin of the servo to the 5V output of the microcontroller.
- Ground Connection: Connect the GND pin of the servo to the GND of the microcontroller.
- Signal Pin: Connect the control signal pin of the servo to a PWM-enabled digital pin on the microcontroller.
Step 2: Writing the Microcontroller Code
The next step involves coding the microcontroller to generate the appropriate PWM signals to control the servo.
Here’s an example code for Arduino:
#include <Servo.h>
Servo myServo;
void setup() {
myServo.attach(9); // Attach servo to PWM pin 9
}
void loop() {
myServo.write(90); // Set servo to 90 degrees
delay(1000); // Wait for 1 second
myServo.write(0); // Set servo to 0 degrees
delay(1000); // Wait for 1 second
}
In this code, the servo motor is set to rotate between 0 degrees and 90 degrees. The write()
function sends the PWM signal, determining the position of the servo.
Step 3: Uploading the Code
Once your code is ready, upload it to your microcontroller using the Arduino IDE or another compatible platform. After uploading, the servo motor should start responding to the PWM signals, rotating to the desired positions.
Microcontroller Choices for Servo Motor Control
There are many microcontrollers suitable for controlling a servo motor. The choice depends on your application requirements:
- Arduino Uno: Ideal for beginners. Easy to program, comes with a wide range of libraries like the
Servo.h
. - ESP32: Offers built-in WiFi and Bluetooth, making it suitable for IoT applications where remote control is required.
- PIC Microcontroller: Suitable for industrial applications requiring high reliability.
Comparison Table
Microcontroller | Features | Suitable For |
---|---|---|
Arduino Uno | Easy to program, Low cost | Basic control |
ESP32 | Built-in WiFi, Multi-core | IoT, Wireless control |
PIC | Robust, Industrial standard | Professional use |
Pros and Cons of Using Microcontrollers for Servo Control
Pros
- Precise Control: Microcontrollers can provide highly precise control over servo motors.
- Flexibility: Different control algorithms can be easily implemented and tested.
- Integration with Sensors: Microcontrollers allow integration with various sensors and feedback systems.
Cons
- Power Supply Limitations: Servo motors can require higher currents that exceed the capacity of typical microcontroller boards.
- Programming Complexity: Requires basic programming knowledge, which can be challenging for beginners.
Common Issues and Troubleshooting Tips
Servo Motor Not Moving
- Check Connections: Verify all connections are secure, especially power and GND.
- Insufficient Power: Ensure the power supply matches the servo motor’s specifications.
- Wrong Pin Configuration: Make sure the PWM pin is correctly defined in your code.
Jittering Movements
- Noise on Signal Line: Use capacitors to filter out noise from the signal lines.
- Overload Issue: Reduce the load on the servo if it’s causing irregular movements.
Motor Moving Incorrectly
- Incorrect PWM Signal: Adjust the pulse width values to make sure they are between 1ms and 2ms.
- Feedback Issues: Check the feedback mechanism; some servos may have defective potentiometers or encoders.
Advanced Topics: Multi-Servo Control
You can control multiple servo motors using a single microcontroller by assigning each servo to a different PWM-enabled pin. Here’s an example:
#include <Servo.h>
Servo servo1;
Servo servo2;
void setup() {
servo1.attach(9);
servo2.attach(10);
}
void loop() {
servo1.write(45); // Move servo1 to 45 degrees
servo2.write(135); // Move servo2 to 135 degrees
delay(2000); // Wait for 2 seconds
}
This code snippet controls two servos with separate angles. It’s essential to avoid overloading the microcontroller’s power supply when using multiple servos.
Conclusion
Controlling a servo motor using a microcontroller can seem daunting at first, but once you understand the basics of PWM and feedback mechanisms, it becomes straightforward. Whether you’re building a simple robotic arm or a sophisticated automation system, mastering servo control opens up a world of possibilities for innovation. The process involves selecting the right components, ensuring appropriate connections, and writing effective code to bring your project to life.
For more detailed guidance and to find servo motors for your projects, consider visiting our Servo Motor Store. We offer a variety of products suitable for every level of automation need.
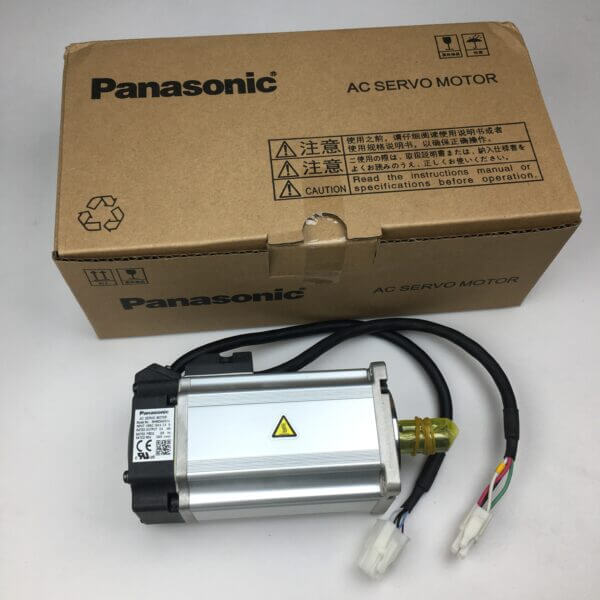
FAQs
1. What microcontroller is best for controlling a servo motor?
The Arduino Uno is a great choice for beginners, while ESP32 is suitable for IoT projects requiring wireless control. For industrial applications, PIC microcontrollers are often preferred.
2. How do I control multiple servo motors with one microcontroller?
You can control multiple servos using different PWM-enabled pins. Libraries like Servo.h
allow you to attach and control multiple servo motors simultaneously.
3. What type of power supply do I need for a servo motor?
It’s recommended to use an external power supply that matches the voltage and current ratings of your servo motor, as microcontrollers may not supply enough current.