Mastering Servo Speed Control with Arduino: A Comprehensive Guide
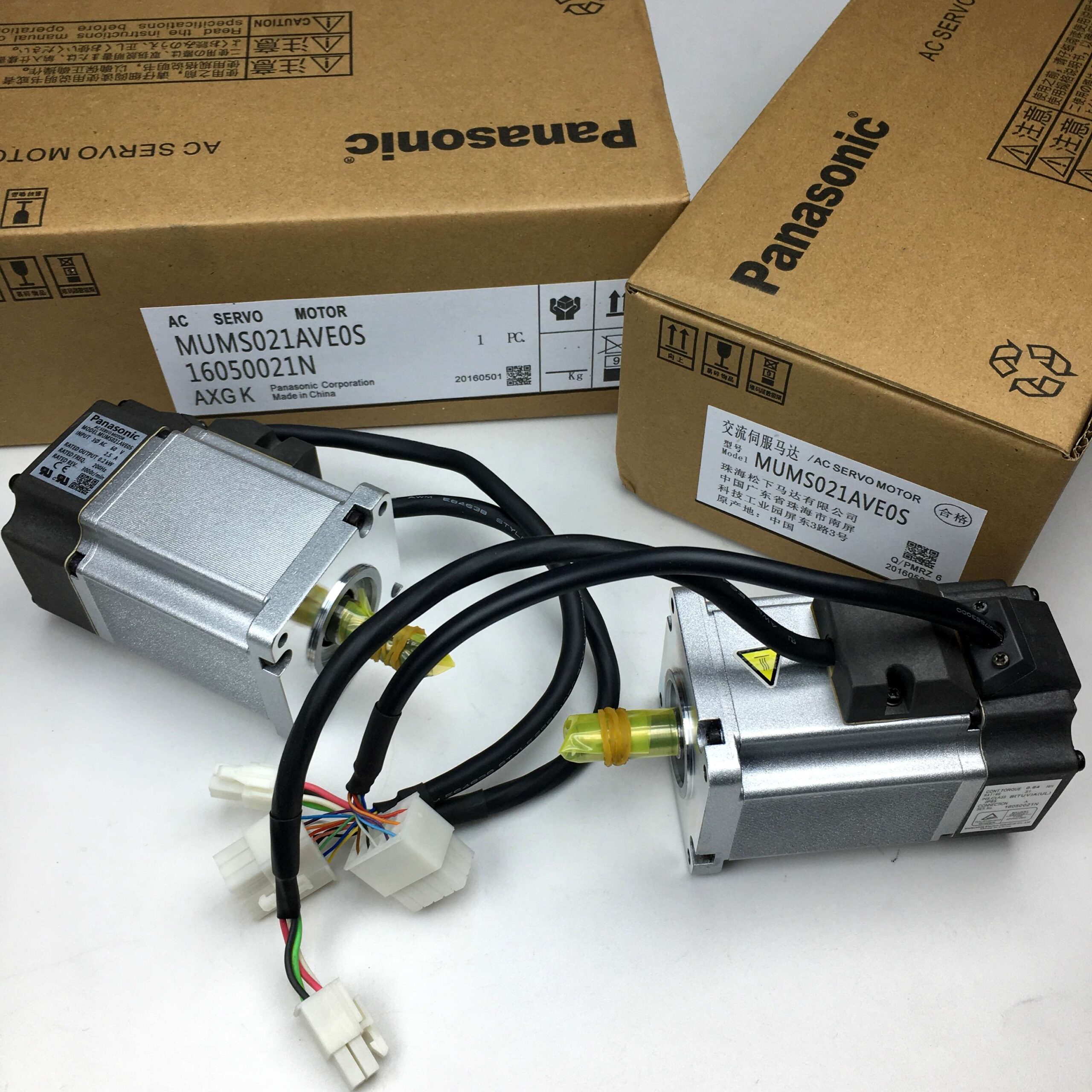
How Does an Arduino Control a Servo Motor?
An Arduino board can control a servo motor by generating a PWM (Pulse Width Modulation) signal. The Arduino IDE includes a built-in Servo library (servo.h) that simplifies the process of controlling servos. You can create a servo object and use simple commands to set the position of the servo.
To use the Servo library, you first need to include it at the beginning of your code with #include <Servo.h>
. Then, you can create a servo object, for example, Servo myservo;
. In the void setup()
function, you attach the servo to a specific pin using myservo.attach(pin);
. The pin should be one of the Arduino’s PWM-capable pins.
Once the servo is attached, you can use the myservo.write(angle);
function to set the position of the servo, where angle
is a value between 0 and 180 degrees. The Servo library automatically generates the appropriate PWM signal to move the servo to the desired position.
What is PWM and How Does it Relate to Servo Speed?
PWM, or Pulse Width Modulation, is a technique used to control the amount of power delivered to a device by rapidly switching the power on and off. The width of the “on” pulse determines the average power delivered. In the context of servos, PWM signals are used to set the position of the servo arm.
While PWM directly controls the position of a servo, it can indirectly influence the speed of a servo motor. By changing the target position in small increments and introducing delays, you can create the effect of varying the servo speed. The smaller the increments and the shorter the delays, the faster the servo will appear to move. The speed of the servo will be determined by how quickly you change the pulse width.
However, it’s important to note that the actual speed of a servo motor is limited by its internal mechanism and the power supply. You can’t make a servo move faster than its maximum rated speed, but you can control how quickly it reaches its target position within that limit. With PWM, you can control the speed within the limits of the servo motor. The speed of the servo motor is affected by the PWM signal, so it is important to know how to control it.
How to Control the Speed of a Servo with Arduino Code
Controlling the speed of a servo with Arduino involves manipulating the position of the servo over time. You can achieve speed control by moving the servo in small increments and adjusting the delay between each movement. Here is a basic example of Arduino code to control a servo‘s speed:
#include <Servo.h>
Servo myservo;
int pos = 0; // variable to store the servo position
void setup() {
myservo.attach(9); // attaches the servo on pin 9 to the servo object
}
void loop() {
// goes from 0 degrees to 180 degrees
// in steps of 1 degree
for (pos = 0; pos <= 180; pos += 1) {
myservo.write(pos); // tell servo to go to position in variable 'pos'
delay(15); // waits 15ms for the servo to reach the position
}
// goes from 180 degrees to 0 degrees
for (pos = 180; pos >= 0; pos -= 1) {
myservo.write(pos); // tell servo to go to position in variable 'pos'
delay(15); // waits 15ms for the servo to reach the position
}
}
In this code, the servo moves from 0 to 180 degrees and back, one degree at a time, with a 15-millisecond delay between each step. By decreasing the delay, you can make the servo move faster, and by increasing it, you can make it move slower.
Advanced Techniques for Servo Speed Control
For more sophisticated servo speed control, you can implement a control loop that adjusts the servo‘s movement based on its current position and the desired speed. This can involve using a PID (Proportional-Integral-Derivative) controller or other control algorithms to achieve smooth and precise servo movement.
Another technique is to use a variable speed profile, where the speed of the servo changes over time. For example, you might want the servo to start slowly, accelerate to a maximum speed, and then decelerate as it approaches the target position. This can be achieved by dynamically adjusting the increment size and delay within the control loop. The speed of a servo motor can be controlled using variable speed.
You can also use external sensors, such as encoders or potentiometers, to provide feedback on the servo‘s actual position and speed. This information can be used to fine-tune the control loop and achieve even more precise control over the servo‘s movement.
Factors Affecting Servo Speed
Several factors can affect the speed of a servo motor:
- Load: A heavier load on the servo arm will generally result in slower movement.
- Power Supply: An inadequate power supply can limit the servo‘s speed and torque. Ensure that your power source can provide enough current for the servo‘s stall current.
- Gearbox: The internal gearbox of the servo determines its speed and torque characteristics. Servos with lower gear ratios will typically be faster but have less torque.
- Control Signal: The quality and precision of the PWM signal from the Arduino can affect the servo‘s performance.
- Temperature: High operating temperatures can reduce the efficiency of the servo motor and affect its speed.
Wiring Diagram for Connecting a Servo to Arduino
Here’s a simple wiring diagram for connecting a servo to an Arduino board:
- Servo Red Wire (VCC): Connect to Arduino 5V pin
- Servo Brown or Black Wire (GND): Connect to Arduino GND pin
- Servo Yellow or Orange Wire (Signal): Connect to Arduino digital pin 9 (or any PWM-capable pin)
You will also need to connect an external power supply suitable for your servo. Make sure the ground of the external power supply is connected to the Arduino‘s ground.
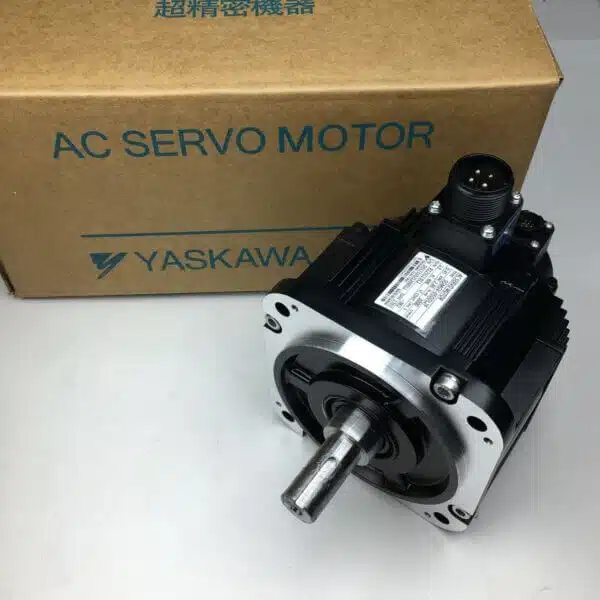
Choosing the Right Servo for Speed Control
When selecting a servo for applications that require speed control, consider the following factors:
- Speed Rating: Check the servo‘s datasheet for its speed rating, typically specified in seconds per 60 degrees of rotation.
- Torque: Ensure that the servo has enough torque to move the intended load at the desired speed.
- Gear Material: Metal gears are generally more durable and can handle higher speeds and loads than plastic gears.
- Digital vs. Analog: Digital servos typically offer better precision and holding power than analog servos.
- Operating Voltage: Most servos operate on 4.8V to 6V, but some can handle higher voltages, which may result in faster speeds.
Common Mistakes to Avoid in Servo Speed Control
Here are some common mistakes to avoid when controlling the speed of a servo motor:
- Exceeding the Servo’s Speed Limit: Trying to make a servo move faster than its maximum rated speed can lead to damage or erratic behavior.
- Insufficient Power Supply: Using a power supply that can’t provide enough current can cause the servo to stall or move slowly.
- Ignoring the Load: Failing to account for the load on the servo can result in slower movement or even damage to the servo.
- Abrupt Changes in Direction: Rapidly changing the servo‘s direction can cause excessive wear and tear.
- Using Incorrect Pulse Widths: Using incorrect pulse widths can cause the servo to jitter or move to the wrong position. You may need to adjust the pulse widths for your particular servo.
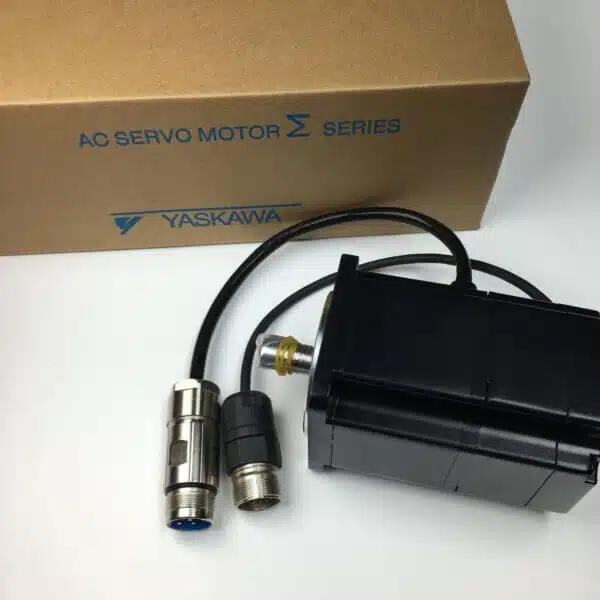
FAQs
1. Can I control the speed of any servo motor with Arduino?
Yes, you can control the speed of most standard servo motors with Arduino by adjusting the pulse width of the PWM signal. However, the degree of control may vary depending on the specific servo model.
2. How can I increase the speed of my servo motor?
You can’t increase the speed beyond the servo‘s maximum rated speed. However, you can ensure it operates at its full potential by using an adequate power supply, reducing the load, and using a servo with a higher speed rating. You may also need to adjust the pulse width.
3. What is the difference between a standard servo and a continuous rotation servo?
A standard servo can rotate to a specific position within a limited range (usually 180 degrees), while a continuous rotation servo can rotate continuously in either direction. Continuous rotation servos are often used for driving wheels in robotic applications.
4. Can I use a motor driver to control the speed of a servo?
No, motor drivers are typically used for controlling DC motors, not servos. Servos have their own built-in control circuitry and are controlled using PWM signals directly from the Arduino.
5. How can I make my servo move more smoothly?
You can achieve smoother servo movement by using smaller increments and adjusting the delay between each movement. Implementing a control loop, such as a PID controller, can also help achieve smoother and more precise servo motion.
6. Why is my servo jittering or not reaching the desired position?
Jittering or inaccurate positioning can be caused by several factors, including an inadequate power supply, incorrect pulse widths, a faulty servo, or electrical noise. Ensure that your servo is properly powered and that you’re using the correct pulse widths for your specific model.
Conclusion
Controlling the speed of a servo motor with Arduino opens up a wide range of possibilities for robotics projects and automation systems. By understanding the principles of PWM, the capabilities of the Servo library, and the factors that affect servo performance, you can achieve precise and dynamic control over your servo‘s movements. Remember to choose the right servo for your application, provide an adequate power supply, and carefully design your control code to achieve the desired speed and accuracy. With the knowledge gained from this guide, you’re well-equipped to tackle your next servo-powered project with confidence.
Key Takeaways
- Servo motors are geared motors that can rotate to a specific angular position, controlled by Pulse Width Modulation (PWM) signals.
- Arduino can control servo speed by adjusting the position in small increments and varying the delay between movements.
- The Servo library in Arduino simplifies the process of controlling servos, allowing you to set the position using
servo.write()
. - PWM controls the average power delivered to the servo by varying the width of the “on” pulse.
- Factors affecting servo speed include load, power supply, gearbox ratio, control signal quality, and temperature.
- Proper material selection for servo components is crucial for wear resistance, friction reduction, and temperature resistance.
- Advanced techniques like control loops and variable speed profiles can achieve more sophisticated servo speed control.
- Common mistakes to avoid include exceeding the servo‘s speed limit, using an insufficient power supply, ignoring the load, and using incorrect pulse widths.
- Choosing the right servo involves considering speed rating, torque, gear material, and operating voltage.
- Regular maintenance, such as checking for wear and ensuring proper lubrication, is essential for optimal servo performance.
Here are some relevant internal links using semantically relevant rich anchor text.